Arduino Mega LCD TFT 320x480 Mandelbrot
26.09.2017
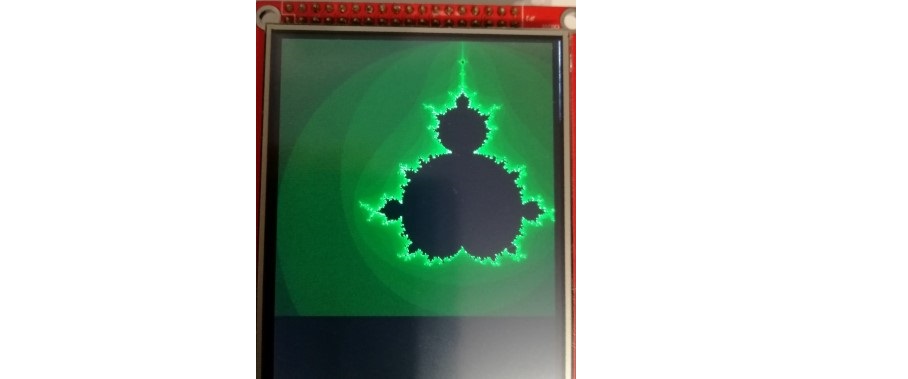
The Mandelbrot set is the set of complex numbers c for which the function ............
more teory here: https://en.wikipedia.org/wiki/Mandelbrot_set
or here: https://mathworld.wolfram.com/MandelbrotSet.html
Used:
3.5 inch TFT LCD touch screen Module for Arduino mega2560 for US $13.49 :
Arduino mega - clone
Code:
Below is procedure to calculate and view mandelbrot set on LCD display. Procedure has 7 parameters.
x0,y0,y1,x1 - start and stop coordinate in mandelbrot set
sx,sy -size of generated picture in pixels
lim - limit number of iteration that are used to compute each pixel. higher limit = better quality = more time
void LCD::mandelbrot(float x0,float y0,float x1, float y1,int16_t sx, int16_t sy,uint16_t lim ){ int16_t limit,counter1,i,j; float cr,ci,zr,zi; limit=lim; setRectangle(0,0,sx-1,sy-1); LCD_Write_COM(RAMWR); float di,dr; //size of delta float sqzr; //square Zr float sqzi; //square Zi di=(y1-y0)/sx; // calculate delta as size/pixel //dr=(x1-x0)/sy; dr=di; cr=x0; //CR for (i=0;i<sy;i++) { cr=cr+dr; ci=y0; for (j=0;j<sx;j++) { ci=ci+di; zr=0.0; zi=0.0; sqzr=zr*zr; sqzi=zi*zi; counter1=0; do {//calculate each pixel zi=zr*zi; zi+=zi; zi+=ci; zr=sqzr-sqzi+cr; sqzr = zr*zr; sqzi = zi*zi; ++counter1; } while ((counter1<=limit) && ((sqzr+sqzi)<=4.0) ); //pixel color transformation uint16_t col = counter1<<5; //GREEN // uint16_t col = (counter1>>1)<<11; //RED // uint16_t col = (counter1>>1); //BLUE if (counter1>63) col=63<<5; //optimalization1 - soft edges if (counter1>=limit) setPixel(BLACK); else setPixel(col); //show pixel } } }
Update1: coloring
code for nice colors
////pixel color transformation //uint16_t col = counter1<<5; //GREEN //// uint16_t col = (counter1>>1)<<11; //RED //// uint16_t col = (counter1>>1); //BLUE //if (counter1>63) col=63<<5; //optimalization1 - soft edges //if (counter1>=limit) setPixel(BLACK); else setPixel(col); //show pixel uint16_t fi=255*counter1/limit; uint8_t cb,cg,cr; if ((fi>=0)&&(fi<32)) {cb=fi;cr=0;cg=0;} if ((fi>=32)&&(fi<64)) {cg=fi-32;cr=0;cb=31;} if ((fi>=64)&&(fi<96)) {cb=95-fi;cg=31;cr=0;} if ((fi>=96)&&(fi<128)) {cr=fi-96;cg=31;cb=0;} if ((fi>=128)&&(fi<160)) {cg=159-fi;cr=31;cb=0;} if ((fi>=160)&&(fi<192)) {cb=fi-160;cg=0;cr=31;} if ((fi>=192)&&(fi<224)) {cg=fi-192;cr=31,cb=31;} if ((fi>=224)&&(fi<256)) {cr=255-fi;cg=31;cb=31;} uint16_t pc= (((cb<<3)&0b11111000)>>3)|(((cg<<3)&0b11111000)<<3)|(((cr<<3)&0b11111000)<<8); if (counter1>=limit) setPixel(BLACK); else setPixel(pc);
Gallery
Download Atmel Studio 7.0 Project