SSD1306 128x64 OLED display with TWI (I2C) text mode only
21.02.2017
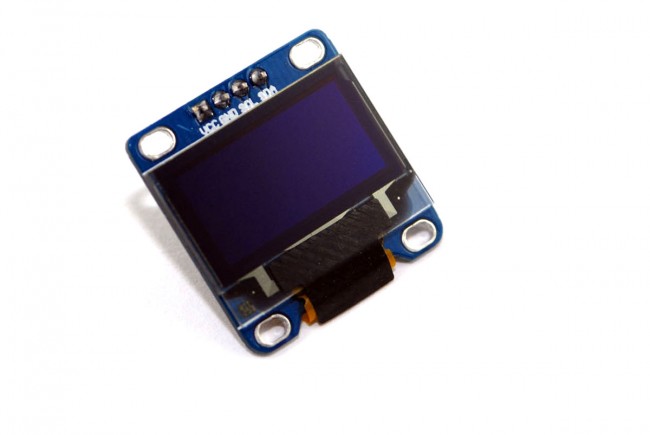
Simple text mode library with 8pix height font.
Used:
128x64 pixel white OLED Display SSD1306 -4 pin connector for 2,47 € from:
Aduino Nano 3.0 Clone for 2,10 € from:
2x 2k2 resistor
Breadboard
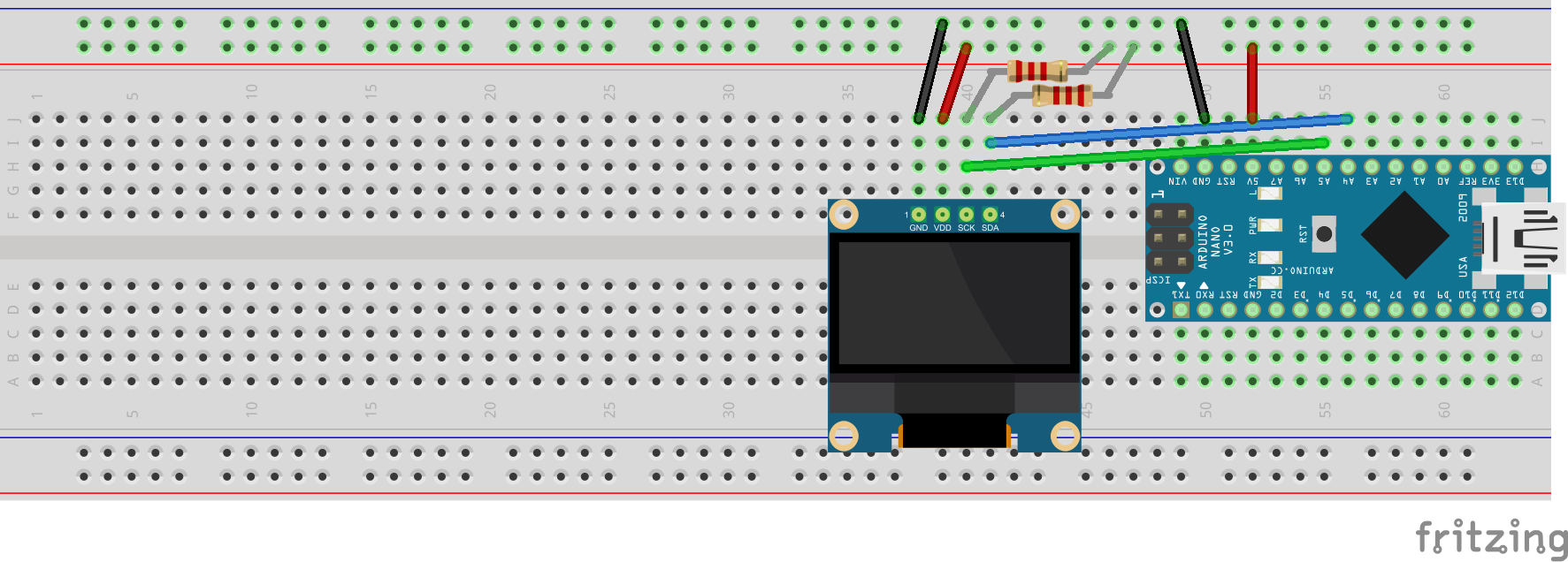
Schematic
Photo
Check connection and communication
#define F_CPU 16000000UL #include <avr/io.h> #include <util/delay.h> #include "My_uart.h" #include "TWI.h" #include <avr/interrupt.h> int main(void) { initUART(); uart_println("TWI test"); TWIInit(); //check all address for (uint8_t i=0;i<128;i++) { if(TWIcheckAdress(i<<1)) { uart_print("device found at 0x"); uart_printh(i<<1); uart_putchar('\n'); } } uart_println("end "); while (1) { } }
Download Atmel Studio 7.0 Project
SSD1306 initalization
void ssd1306_init(){ // Init sequence for 128x64 OLED module ssd1306_command(SSD1306_DISPLAYOFF); // 0xAE ssd1306_command(SSD1306_SETDISPLAYCLOCKDIV); // 0xD5 ssd1306_command(0x80); // the suggested ratio 0x80 ssd1306_command(SSD1306_SETMULTIPLEX); // 0xA8 ssd1306_command(0x3F); ssd1306_command(SSD1306_SETDISPLAYOFFSET); // 0xD3 ssd1306_command(0x0); // no offset ssd1306_command(SSD1306_SETSTARTLINE); // line #0 ssd1306_command(SSD1306_CHARGEPUMP); // 0x8D ssd1306_command(0x14); // using internal VCC ssd1306_command(SSD1306_MEMORYMODE); // 0x20///// ssd1306_command(0x00); // 0x00 horizontal addressing ssd1306_command(SSD1306_SEGREMAP | 0x1); // rotate screen 180 ssd1306_command(SSD1306_COMSCANDEC); // rotate screen 180 ssd1306_command(SSD1306_SETCOMPINS); // 0xDA ssd1306_command(0x12); ssd1306_command(SSD1306_SETCONTRAST); // 0x81 ssd1306_command(0xCF); ssd1306_command(SSD1306_SETPRECHARGE); // 0xd9 ssd1306_command(0xF1); ssd1306_command(SSD1306_SETVCOMDETECT); // 0xDB ssd1306_command(0x40); ssd1306_command(SSD1306_DISPLAYALLON_RESUME); // 0xA4 ssd1306_command(SSD1306_NORMALDISPLAY); // 0xA6 ssd1306_command(SSD1306_DISPLAYON); //switch on OLED }
Display after initialization
Sample text output
#define F_CPU 16000000UL #include <avr/io.h> #include <util/delay.h> #include "My_uart.h" #include "TWI.h" #include <avr/interrupt.h> #include "font.h" #include "SSD1306.h" int main(void) { initUART(); uart_println("TWI test"); TWIInit(); //check all address ssd1306_init(); ssd1306_clear(); for (uint8_t i=32; i<124;i++) { ssd1306_char(i); } ssd1306_print(" SSD1306 OLED 128x64 Display "); ssd1306_print(" Ketonk 2017 ;-) "); for (uint8_t i=0; i<127;i++) ssd1306_data(0b11000000); while (1) { } }
Download project